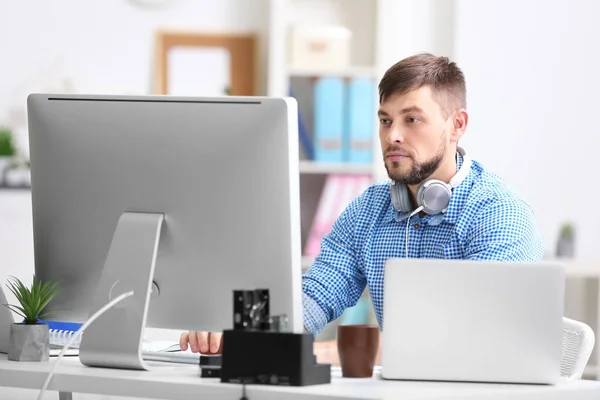
Delivering applications with agility, scalability, and resilience is paramount. Microservices architecture has emerged as a popular approach to achieve these goals. Let’s explore how Spring Boot and Spring Cloud can be leveraged to develop and manage microservices, enabling efficient and robust application development.
Understanding Microservices Architecture
Microservices architecture is a design pattern where an application is composed of loosely coupled, independently deployable services. Each service is responsible for a specific business functionality and operates independently, communicating with other services through well-defined APIs.
Key benefits of microservices architecture include:
- Scalability: Each service can be scaled independently based on its demand, allowing for efficient resource utilization.
- Flexibility: Developers can work on different services simultaneously, utilizing various technologies and frameworks that best suit each service’s requirements.
- Resilience: Failure in one service does not affect the entire application, ensuring better fault tolerance and uptime.
- Maintainability: Easier to manage and maintain due to smaller, focused codebases.
Spring Boot: Simplifying Microservices Development
Spring Boot, part of the larger Spring Framework ecosystem, simplifies the development of microservices by providing production-ready defaults for application configuration and components. It facilitates building stand-alone, production-grade Spring-based applications with minimal effort.
Key features of Spring Boot include:
- Auto-configuration: Automatically configures application components based on the libraries on the classpath.
- Standalone: Enables the creation of executable JAR or WAR files for easy deployment.
- Opinionated defaults: Provides sensible defaults, reducing the need for manual configuration.
Spring Cloud: Managing Microservices Architecture
Spring Cloud, another component of the Spring ecosystem, focuses on building robust cloud-native applications. It offers a set of tools and frameworks to assist in building, deploying, and managing microservices applications.
Key features of Spring Cloud include:
- Service Discovery and Registration: Eureka, Consul, or ZooKeeper allow services to discover and register with each other dynamically.
- Load Balancing: Ribbon provides client-side load balancing for service-to-service communication.
- Circuit Breaker Pattern: Hystrix helps in creating resilient applications by implementing the circuit breaker pattern.
- API Gateway: Spring Cloud Gateway provides a flexible and powerful way to route and filter requests to services.
Building Microservices with Spring Boot and Spring Cloud
Let’s outline the steps to build microservices using Spring Boot and Spring Cloud:
1. Define Service Boundaries
Identify the various functionalities of your application and define the boundaries for each service. Each service should handle a specific business capability.
2. Create Spring Boot Projects
Use Spring Boot to create individual Spring Boot projects for each microservice. This involves setting up the necessary dependencies, configurations, and logic for each service.
3. Implement Business Logic
Develop the business logic within each service. Ensure that each service is responsible for a distinct aspect of the application’s functionality.
4. Enable Service Discovery
Configure service discovery using Spring Cloud Eureka or another service registry. Services should register themselves upon startup and discover other services dynamically.
5. Implement Communication Between Services
Utilize Spring Cloud libraries like Feign or RestTemplate for inter-service communication. Implement communication patterns like circuit breakers to handle failures gracefully.
6. Implement API Gateway
Create an API Gateway using Spring Cloud Gateway to route requests to the appropriate microservice. This provides a single entry point for clients and simplifies the architecture.
7. Ensure Data Consistency
Implement data consistency strategies, such as event sourcing or distributed transactions, to ensure data integrity across microservices.
8. Monitor and Manage Microservices
Utilize Spring Cloud Sleuth and Zipkin for distributed tracing and monitoring. Set up logging and monitoring systems to track the health and performance of microservices.
9. Deploy and Scale Microservices
Deploy microservices individually and scale them as needed to handle varying loads. Utilize containerization and orchestration tools like Docker and Kubernetes for efficient deployment and scaling.
Simplifying Microservices Delivery
Microservices architecture offers a scalable and flexible approach to application development. Spring Boot and Spring Cloud provide a comprehensive set of tools and frameworks to simplify the development, deployment, and management of microservices. By following best practices and leveraging the capabilities of these technologies, you can build robust, resilient, and highly scalable applications tailored to meet modern business needs.